[Angular | Capacitor] Interact With Your Native Calendar
Recently I had do to implement a simple task: When the user presses the 'save appointment' button, the app should add this event to the native calendar. My first thought was saving an .ical file and open it, till i recognized that this isn't the most user friendly way.
After a little search I found a really great github project which makes this task a no brainer.
Install & Setup
npm i cordova-plugin-calendar
npm i @awesome-cordova-plugins/calendar
npx cap sync
If you sync your iOS project you may get some warnings. Just do what they say ;-)
[warn] Configuration required for cordova-plugin-calendar.
Add the following to Info.plist:
<key>NSCalendarsUsageDescription</key>
<string>$CALENDAR_USAGE_DESCRIPTION</string>
[warn] Configuration required for cordova-plugin-calendar.
Add the following to Info.plist:
<key>CFBundleLocalizations</key>
<array>
<string>en</string>
<string>de</string>
<string>nl</string>
<string>fr</string>
<string>it</string>
<string>pt-BR</string>
</array>
Switch to your angular module and add Calendar to your providers.
import { Calendar } from '@awesome-cordova-plugins/calendar/ngx';
@NgModule({
...
providers: [Calendar],
})
Nice - setup should be done!
Exploring the Calendar API
Now switch to the component where you need to interact with the phones calendar and add the Calendar Plugin to your constructor.
import { Calendar } from '@awesome-cordova-plugins/calendar/ngx';
export class .... {
constructor(private calendar: Calendar) {}
}
Let us explore the declared calendar property with some examples.
Create Event
this.addEventResult = this.calendar.createEventInteractively(
'My Hero Event', // title
'Hero Garden', // location
undefined, // notes
new Date(), // start date
undefined // end date
);
Opens the "Create Event" dialog with pre-filled data from the built-in Calendar app.
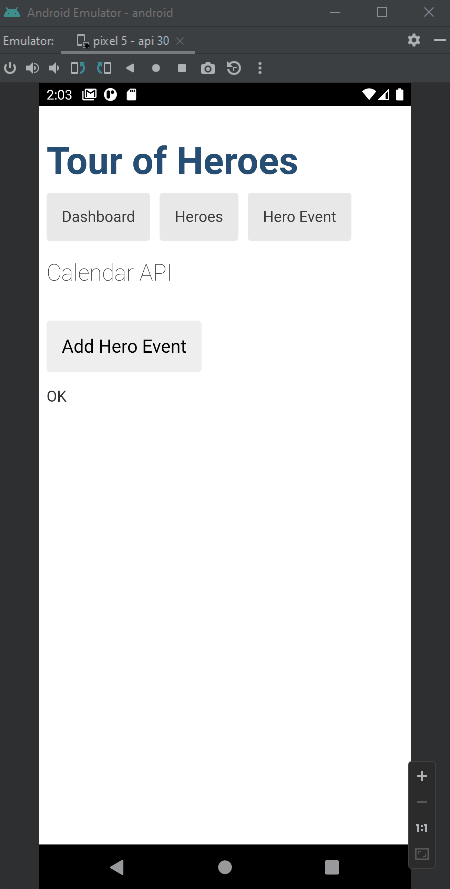
List Calendars
this.availableCalendar = this.calendar.listCalendars();
On Android you will get something like this as a result.
[
{
"id": "1",
"name": "yesreply@georghoeller.dev",
"displayname": "yesreply@georghoeller.dev",
"isPrimary": true
}
]
Edit, Delete, ...
The plugin can do much more - for the full feature list checkout the projects github page: Github
Georg Hoeller Newsletter
Join the newsletter to receive the latest updates in your inbox.